Sending Emails Using SMTP and Maileroo API in Java
Learn to send emails in Java using Maileroo! This guide covers SMTP and Maileroo Email API, from setup to sample code for sending notifications, confirmations, or newsletters. Simplify email integration and enhance user communication with easy-to-follow steps and examples.
If you’re building a Java application that needs to send notifications, welcome messages, confirmations, or newsletters, having email functionality is critical. In this guide, we’ll be exploring two different methods to send emails in Java using Maileroo:
- SMTP (Simple Mail Transfer Protocol)
- Maileroo Email API
We’ll start by creating our development environment from scratch. Next, we’ll begin to configure our Maileroo Account (such as verifying your domain and collecting credentials). Finally, we’ll write sample code for each approach. By the end of this tutorial, you’ll be able to send emails confidently to your users.
What is Maileroo?
Maileroo is a straightforward email service that provides two main methods of sending emails:
- SMTP: A “traditional” email protocol
- Maileroo Email API: A modern HTTP-based endpoint for sending messages
Using Maileroo you can simplify your email-sending process and reduce complexity compared to managing your own personal mail server.
Creating Maileroo Account
Let's create a free Maileroo account by visiting Register | Maileroo and verifying your domain. This process only takes a couple of minutes and is very simple.
- After signing up, you’ll receive a verification email. Click the link in your email to verify your account.
- Once verified, log in to your account.
- From the dashboard, choose SMTP Relay to begin.
Creating an SMTP Account
To send emails using SMTP, we first need to set up the necessary credentials.
- Go to Domains | Maileroo and click on Overview.
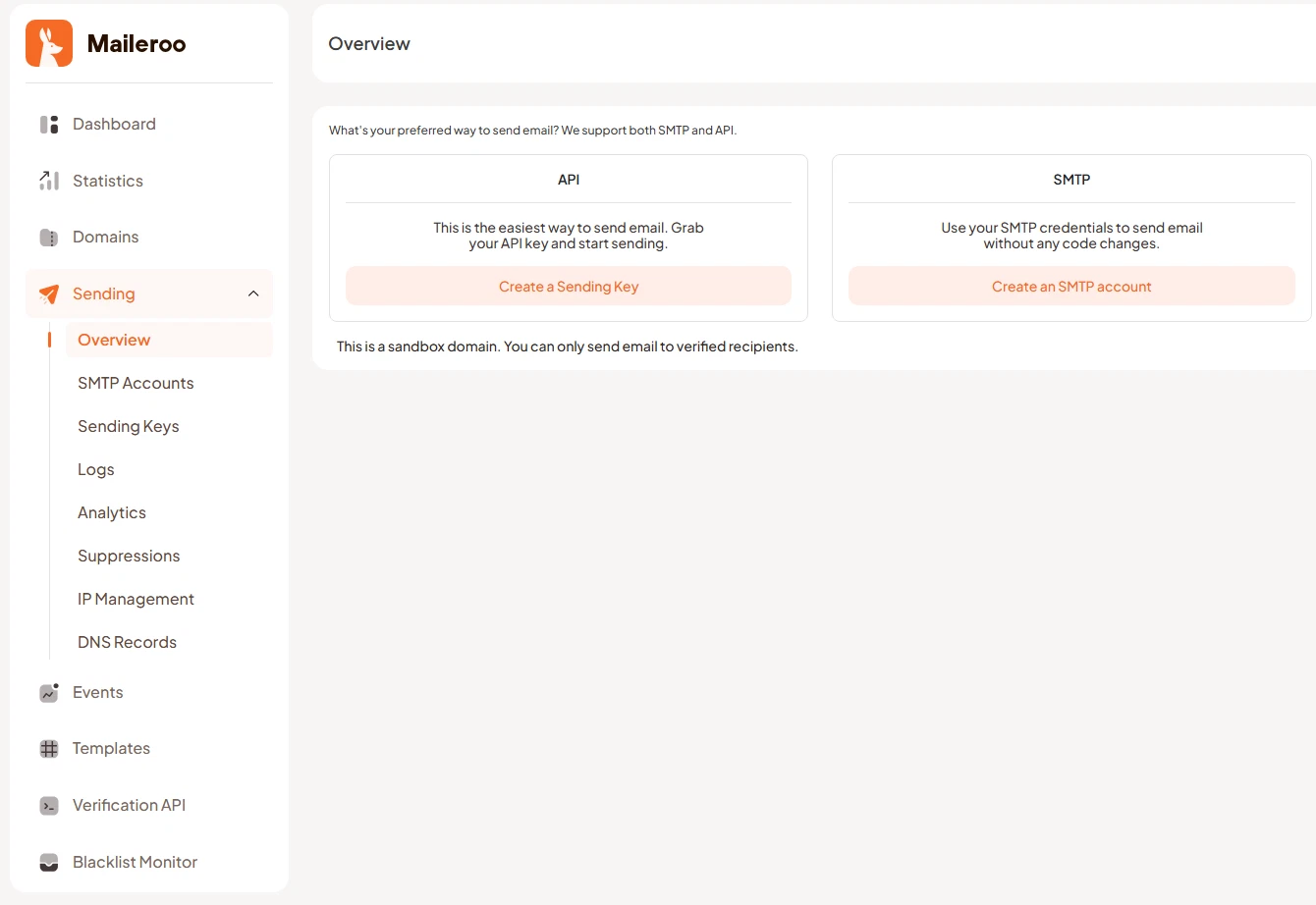
- Click on Create an SMTP Account and then New Account
- Add an alias for the email address you’ll use to send emails.
That's it, your SMTP credentials are now ready to use.
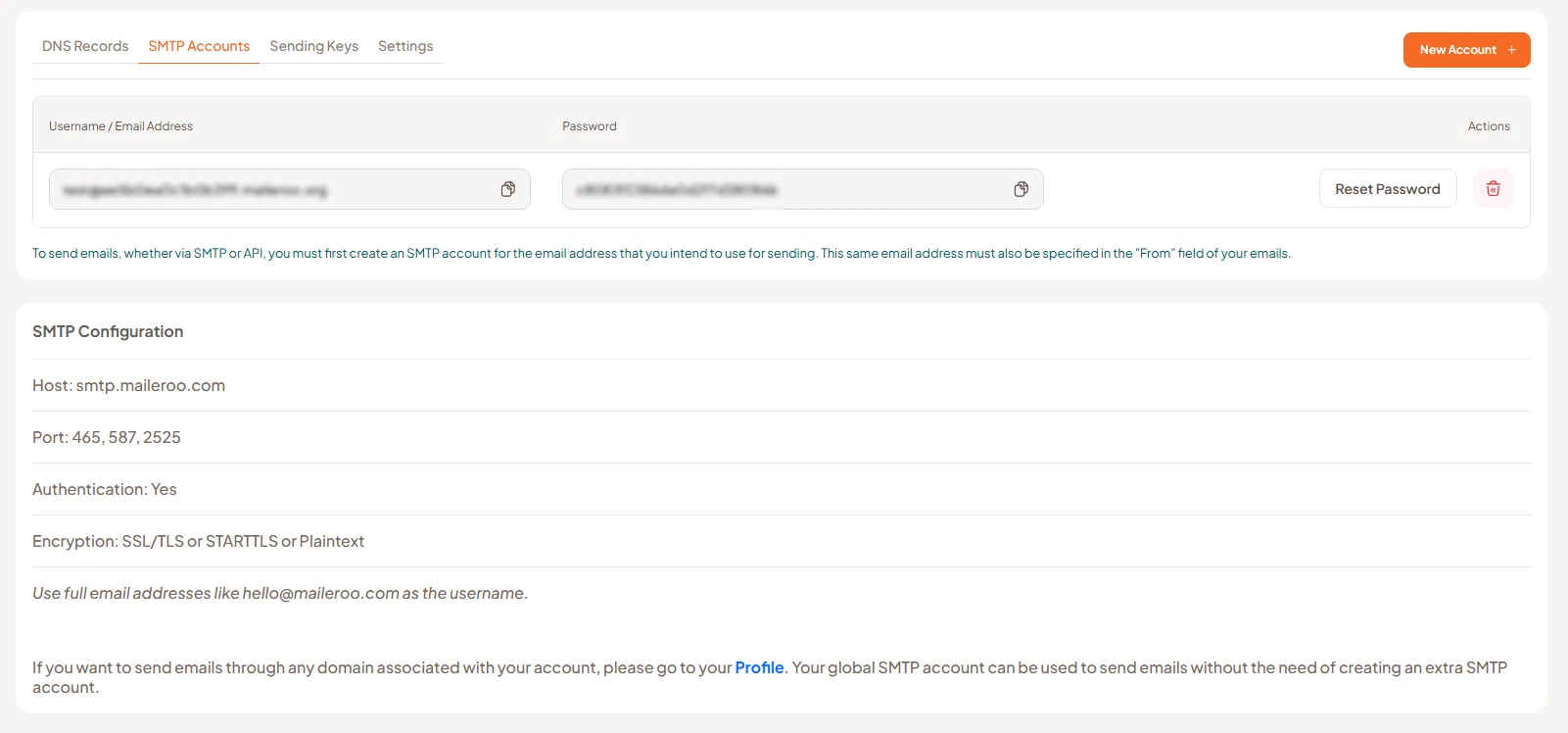
Creating a Sending Key for API Use
If you prefer to send emails using the Maileroo Email API, you’ll need an API key for authentication. Here’s how to create it:
- Navigate to Overview in your domain settings and click on Create a Sending Key, then New Sending Key.
- Provide an identifier (you can use any name you like).

We can now use this API key to send emails.
Adding Authorized Recipients
Before we can send any emails, the recipient's email address must be added to the Authorized Recipients list.
- In the Overview section of your domain, check the Authorized Recipients panel on the right-hand side.
- Add the recipient's email address.
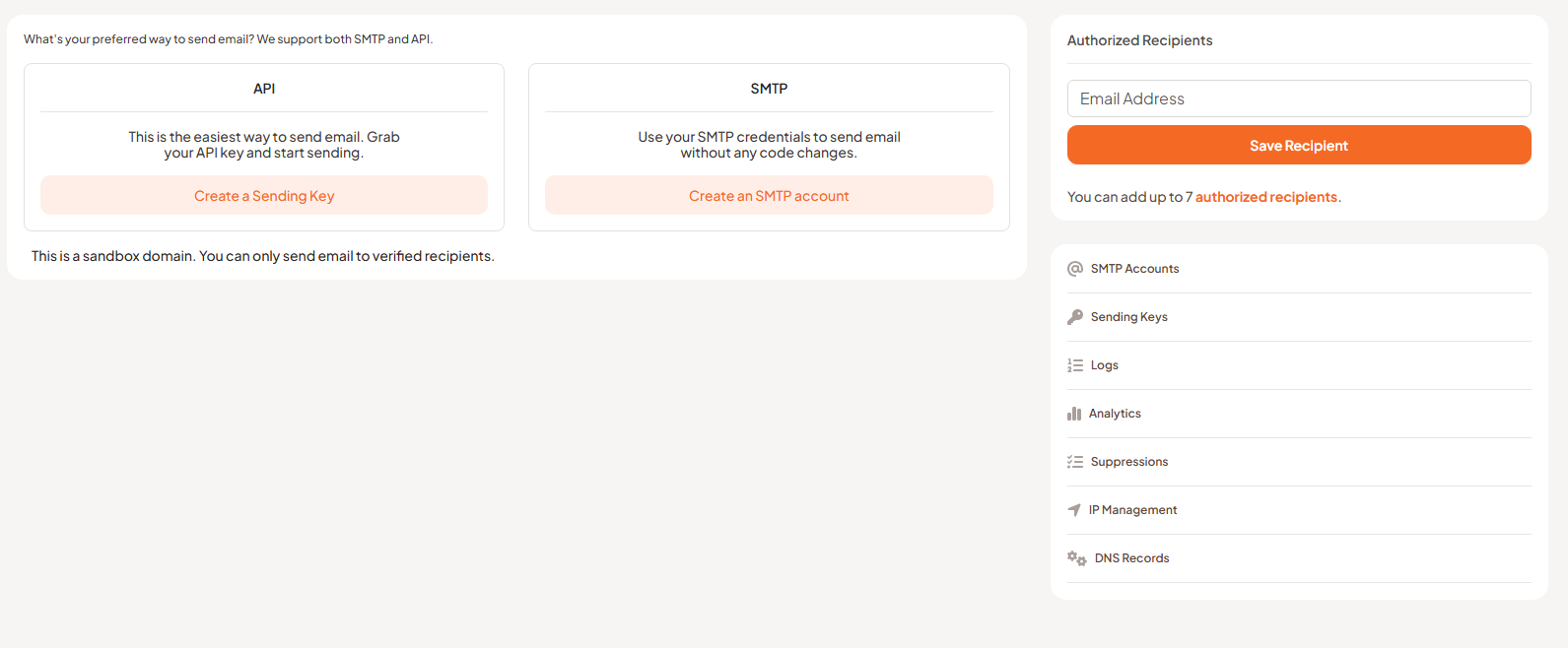
With your environment set up ready and Maileroo account, let's start working on sending emails with Maileroo!
Getting Started
Before writing any code, let’s first configure a fresh Java project
1. Installing Java
Make sure you have Java 8 or later installed. To check your version, run the following command in the terminal:
java -version
If you see a version below 8, upgrade before proceeding. Java 11 or later is recommended for newer language features and better support.
2. Using a Build Tool (Recommended)
While you can compile individual .java
files by hand, Maven and Gradle are the most common tools for managing dependencies and builds.
Maven and Gradle are not typically pre-installed with Java. Instead you will have to install them separately for managing dependencies:
- Maven Installation:
- Download from https://maven.apache.org/download.cgi, unzip, and add
mvn
to your systemPATH
. - Or use a package manager (e.g.,
brew install maven
on macOS orsudo apt-get install maven
on Ubuntu).
- Download from https://maven.apache.org/download.cgi, unzip, and add
- Gradle Installation:
- Download from https://gradle.org/releases/, unzip, and add
gradle
to yourPATH
. - Or install via a package manager (e.g.,
brew install gradle
on macOS orsudo apt-get install gradle
on Ubuntu).
- Download from https://gradle.org/releases/, unzip, and add
Once installed, we can add libraries like Jakarta Mail or OkHttp by editing our pom.xml
(for Maven) or build.gradle
(for Gradle).
3. Folder Structure
We will create a folder structure similar to the following:
email-project/
├── src
│ └── main
│ └── java
│ └── SmtpSender.java
│ └── ApiSender.java
└── pom.xml (if using Maven) or build.gradle (if using Gradle)
We will place our .java
files under src/main/java
.
You can create this structure manually, but using an build tool is better and safer for the project. It also simplifies updates and migrations later.
- Maven:
mvn archetype:generate
- Gradle:
gradle init --type java-application
These generates a barebone project with src/main/java
and pom.xml (Maven) or build.gradle (Gradle)
.
Either approach works fine. The key is to keep your source code in a src/main/java
directory and your build configuration file (pom.xml
or build.gradle
) at the root.
Sending Emails via SMTP
SMTP (Simple Mail Transfer Protocol) is the standard protocol used for sending emails over the Internet.
It’s a reliable and widely-used way to send emails from one place to another, whether for personal use or in applications that send notifications or messages. SMTP works behind the scenes to ensure emails are properly delivered, making it easy to connect to most email services without needing special tools or software.
In Java, the Jakarta Mail (formerly JavaMail) library is commonly used to send emails via SMTP. Let's start by adding it to our project.
Adding Jakarta Mail
- Using Maven:
In your pom.xml
(located in the project root)
<dependencies>
<dependency>
<groupId>com.sun.mail</groupId>
<artifactId>jakarta.mail</artifactId>
<version>2.1.2</version>
</dependency>
</dependencies>
You can then run:
mvn clean install
or
mvn compile
to download and integrate the dependency.
- Using Gradle:
In your build.gradle
:
dependencies {
implementation 'com.sun.mail:jakarta.mail:2.1.2'
}
Then run:
gradle build
to fetch the dependency.
Next create a file named SmtpSender.java
in the src/main/java
. And then we can use the following code snippet to send the email:
import jakarta.mail.*;
import jakarta.mail.internet.InternetAddress;
import jakarta.mail.internet.MimeMessage;
import java.util.Properties;
public class SmtpSender {
// Replace these with actual values from your Maileroo account
private static final String SMTP_HOST = "smtp.maileroo.com";
private static final int SMTP_PORT = 587;
private static final String SMTP_USERNAME = "your-smtp-username";
private static final String SMTP_PASSWORD = "your-smtp-password";
private static final String FROM_NAME = "My App"; // The name shown in the "From" field
private static final String TO_ADDRESS = "someone@example.com"; // Must be on your authorized list
public static void main(String[] args) {
sendEmail();
}
private static void sendEmail() {
// 1. Mail server properties
Properties props = new Properties();
props.put("mail.smtp.auth", "true");
props.put("mail.smtp.starttls.enable", "true");
props.put("mail.smtp.host", SMTP_HOST);
props.put("mail.smtp.port", String.valueOf(SMTP_PORT));
// 2. Create a mail session using your credentials
Session session = Session.getInstance(props, new Authenticator() {
@Override
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication(SMTP_USERNAME, SMTP_PASSWORD);
}
});
try {
Message message = new MimeMessage(session);
message.setFrom(new InternetAddress(SMTP_USERNAME, FROM_NAME));
message.setRecipient(Message.RecipientType.TO, new InternetAddress(TO_ADDRESS));
message.setSubject("SMTP Mail with Maileroo");
message.setText("Hello! This email was sent using Maileroo's SMTP service.");
Transport.send(message);
System.out.println("Email sent successfully via SMTP!");
} catch (Exception e) {
System.out.println("Could not send email: " + e.getMessage());
e.printStackTrace();
}
}
}
To run the code you can use:
- Maven:
mvn compile
mvn exec:java -Dexec.mainClass="SmtpSender"
- Gradle:
gradle run
*If you receive any authentication or connection errors, verify your SMTP credentials in the Maileroo dashboard. You can also check the logs for more debugging information.
Sending Emails with Maileroo Email API
If you’d like to avoid traditional SMTP configuration, the Maileroo Email API lets you send messages by making a simple HTTP POST request. This method is especially convenient if you’re comfortable with REST APIs or if your application already communicates with external services in a similar manner
In Java, the OkHttp library is a popular choice for making HTTP requests, known for its simplicity, performance, and support for modern features like connection pooling and interceptors. We will be using it in this project.
Adding OkHttp
- Using Maven:
<dependencies>
<dependency>
<groupId>com.squareup.okhttp3</groupId>
<artifactId>okhttp</artifactId>
<version>4.11.0</version>
</dependency>
</dependencies>
- Using Gradle:
dependencies {
implementation 'com.squareup.okhttp3:okhttp:4.11.0'
}
Sending a Simple Email via the API
Create a file named ApiSender.java
in your src/main/java
folder and the following code:
import okhttp3.*;
import java.io.IOException;
public class ApiSender {
private static final String MAILEROO_API_KEY = "your-maileroo-api-key";
private static final String TO_ADDRESS = "someone@example.com"; // Must be authorized in Maileroo
public static void main(String[] args) {
sendEmail();
}
private static void sendEmail() {
OkHttpClient client = new OkHttpClient();
// 1. Build the request body
MultipartBody requestBody = new MultipartBody.Builder()
.setType(MultipartBody.FORM)
.addFormDataPart("from", "Areeb <myemail@maileroo.org>") // Replace with your Maileroo email
.addFormDataPart("to", TO_ADDRESS)
.addFormDataPart("subject", "Maileroo API!")
.addFormDataPart("plain", "This is a test email sent using Maileroo's Email API.")
.build();
// 2. Build the request
Request request = new Request.Builder()
.url("https://smtp.maileroo.com/send")
.addHeader("X-API-Key", MAILEROO_API_KEY)
.post(requestBody)
.build();
try (Response response = client.newCall(request).execute()) {
if (response.isSuccessful()) {
System.out.println("Email sent successfully: " + response.body().string());
} else {
System.out.println("Failed to send email: " + response.body().string());
}
} catch (IOException e) {
System.out.println("Error sending email: " + e.getMessage());
e.printStackTrace();
}
}
}
Sending HTML Emails
You can also send HTML content just by changing the form parameter from plain
to html
:
.addFormDataPart("html", "<h1>Hello!</h1><p>This is an HTML email.</p>")
For multi-part emails (containing both plain text and HTML), you can include both plain
and html
parts and maileroo will automatically combine them.
Customizing the Email Name
You can also customize the sender’s name and format it in different ways:
example@maileroo.com
"Name" <example@maileroo.com>
<example@maileroo.com>
Update the from
field in your email data with the new format.
Using Templates
If you want to take advantage of templates (for example, using placeholder variables like {{firstName}}
in your message and sending bulk messages with personalized name), you can create a template in your Maileroo dashboard and note its template ID.
Here's the code snippet using template to send mail:
import okhttp3.*;
import java.io.IOException;
public class TemplateSender {
private static final String MAILEROO_API_KEY = "your-maileroo-api-key";
private static final String TEMPLATE_ID = "123"; // Replace with your actual template ID
public static void main(String[] args) {
sendTemplateEmail();
}
private static void sendTemplateEmail() {
OkHttpClient client = new OkHttpClient();
// Provide the data needed to fill placeholders in your template
String templateDataJson = "{\"firstName\": \"Alice\"}";
MultipartBody requestBody = new MultipartBody.Builder()
.setType(MultipartBody.FORM)
.addFormDataPart("from", "My App <myemail@maileroo.org>") // Replace with your maileroo email
.addFormDataPart("to", "alice@example.com")
.addFormDataPart("subject", "Welcome!")
.addFormDataPart("template_id", TEMPLATE_ID)
.addFormDataPart("template_data", templateDataJson)
.build();
Request request = new Request.Builder()
.url("https://smtp.maileroo.com/send-template")
.addHeader("X-API-Key", MAILEROO_API_KEY)
.post(requestBody)
.build();
try (Response response = client.newCall(request).execute()) {
if (response.isSuccessful()) {
System.out.println("Template email sent: " + response.body().string());
} else {
System.out.println("Template send failed: " + response.body().string());
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Here:
template_id
matches the template you created in Maileroo.template_data
is a JSON string that fills placeholders such as{{firstName}}
.
Wrapping Up
We’ve now covered both methods for sending emails using SMTP and the Maileroo Email API.
You’re now ready to send emails in Java effortlessly with Maileroo. Whether you choose SMTP or the Email API, Maileroo makes it easy to integrate email functionality into your applications.
Start using it today to enhance your communication with users! For more help and examples, visit the official Maileroo Documentation.