Sending Emails Using SMTP and Maileroo API in Python
Automate email sending with Python using SMTP or the Maileroo API for scalable, reliable delivery. Simplify notifications, confirmations, or campaigns with easy integration and advanced features.
Email communication is an essential part of both personal and professional life, serving as a cornerstone for staying connected and exchanging information. As developers, we often encounter scenarios where automating email sending becomes crucial—be it for sending notifications, confirmations or even birthday wishes.
Thankfully, Python makes this process incredibly approachable. With its built-in support for SMTP (Simple Mail Transfer Protocol) and the flexibility offered by modern APIs like Maileroo, you can seamlessly integrate email functionality into your applications.
In this guide, we’ll explore how to send emails using two practical methods:
- The classic SMTP for sending emails.
- The Maileroo API, which provides a more easy and scalable approach for email delivery.
Getting Started
First, let's check if Python is installed on your system. You can do this by opening a terminal or command prompt and running:
python --version
If Python is installed, this command will display the version number. If not, you’ll need to install Python from the official Python website. Make sure to download and install the latest version compatible with your operating system.
Next, let’s set up a clean workspace for the project:
mkdir email_automation;
cd email_automation;
Inside this folder, it’s a good practice to create a virtual environment so that we can isolate the project dependencies. Run the following commands to create and activate the virtual environment:
python -m venv venv
On Windows, activate it with:
venv\Scripts\activate
On macOS or Linux, activate it with:
source venv/bin/activate
Once the virtual environment is activated, we can install the required packages. For this project, we’ll be using Python’s built-in smtplib
module for SMTP functionality and the requests
library to interact with the Maileroo API. Install requests
by running:
pip install requests
Now let's create our Maileroo account!
Creating Maileroo Account
Let's create a free Maileroo account by visiting Register | Mail and verifying your domain. This process only takes a couple of minutes and is very simple.
- After signing up, you’ll receive a verification email. Click the link in your email to verify your account.
- Once verified, log in to your account.
- From the dashboard, choose SMTP Relay to begin.
Creating an SMTP Account
To send emails using SMTP, we first need to set up the necessary credentials.
Go to Domains | Maileroo and click on Overview.
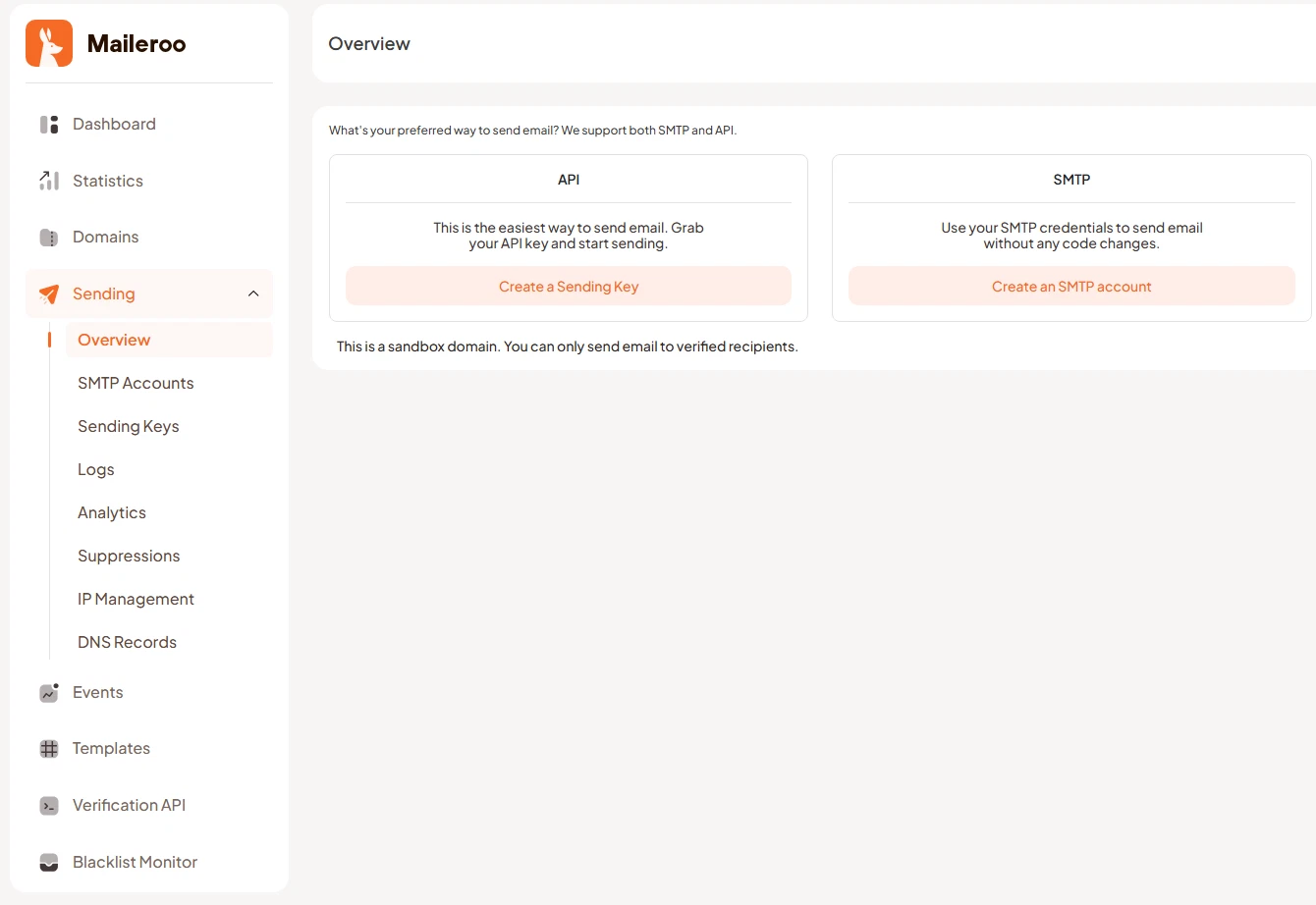
Click on Create an SMTP Account and then New Account.
Add an alias for the email address you’ll use to send emails.
That's it, your SMTP credentials are now ready to use.
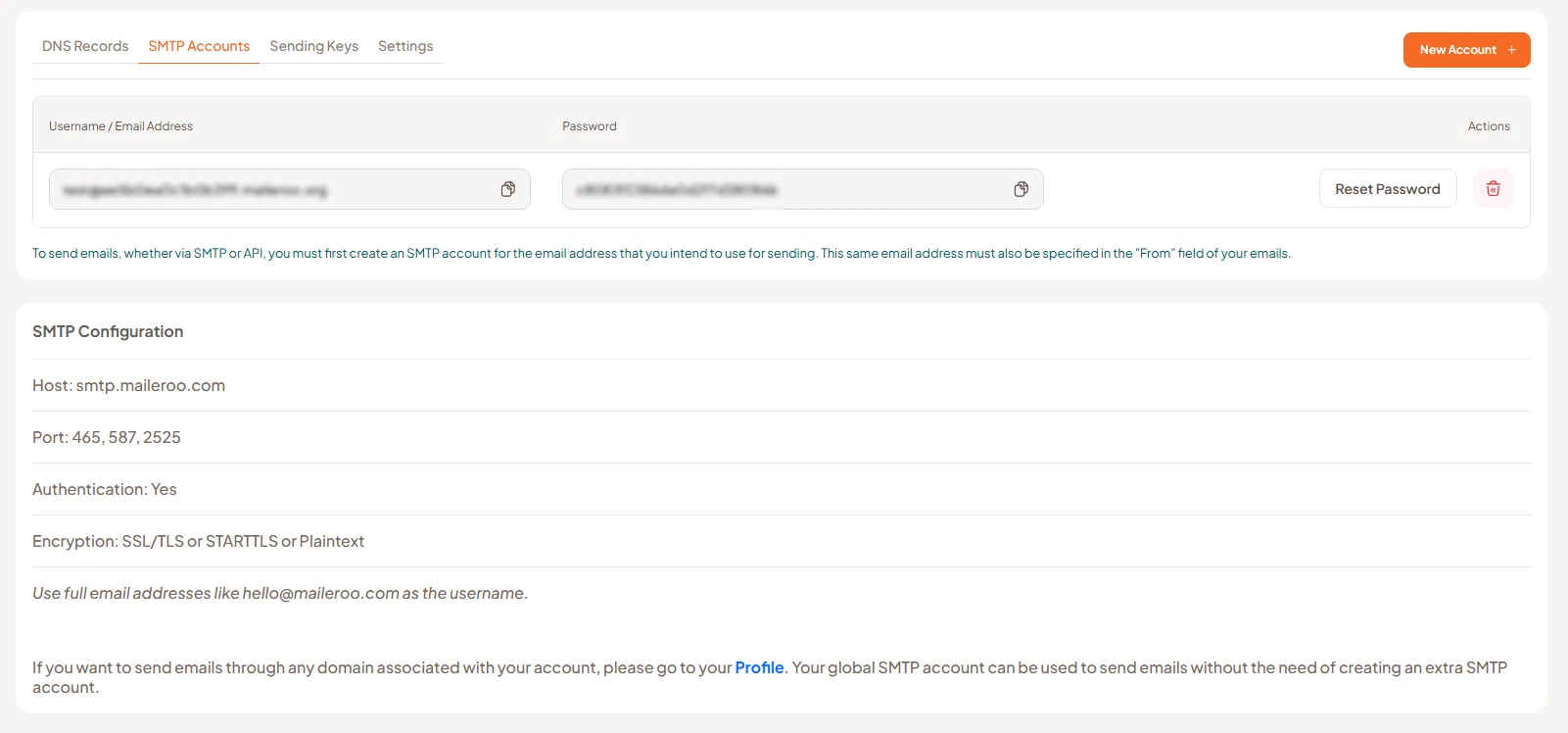
Creating a Sending Key for API Use
If you prefer to send emails using the Maileroo Email API, you’ll need an API key for authentication. Here’s how to create it:
- Navigate to Overview in your domain settings and click on Create a Sending Key, then New Sending Key.
- Provide an identifier (you can use any name you like).

We can now use this API key to send emails.
Adding Authorized Recipients
Before we can send any emails, the recipient's email address must be added to the Authorized Recipients list. You can ignore this step if you have your own domain as this is only required for sandbox (testing) domains offered by Maileroo.
- In the Overview section of your domain, check the Authorized Recipients panel on the right-hand side.
- Add the recipient's email address.
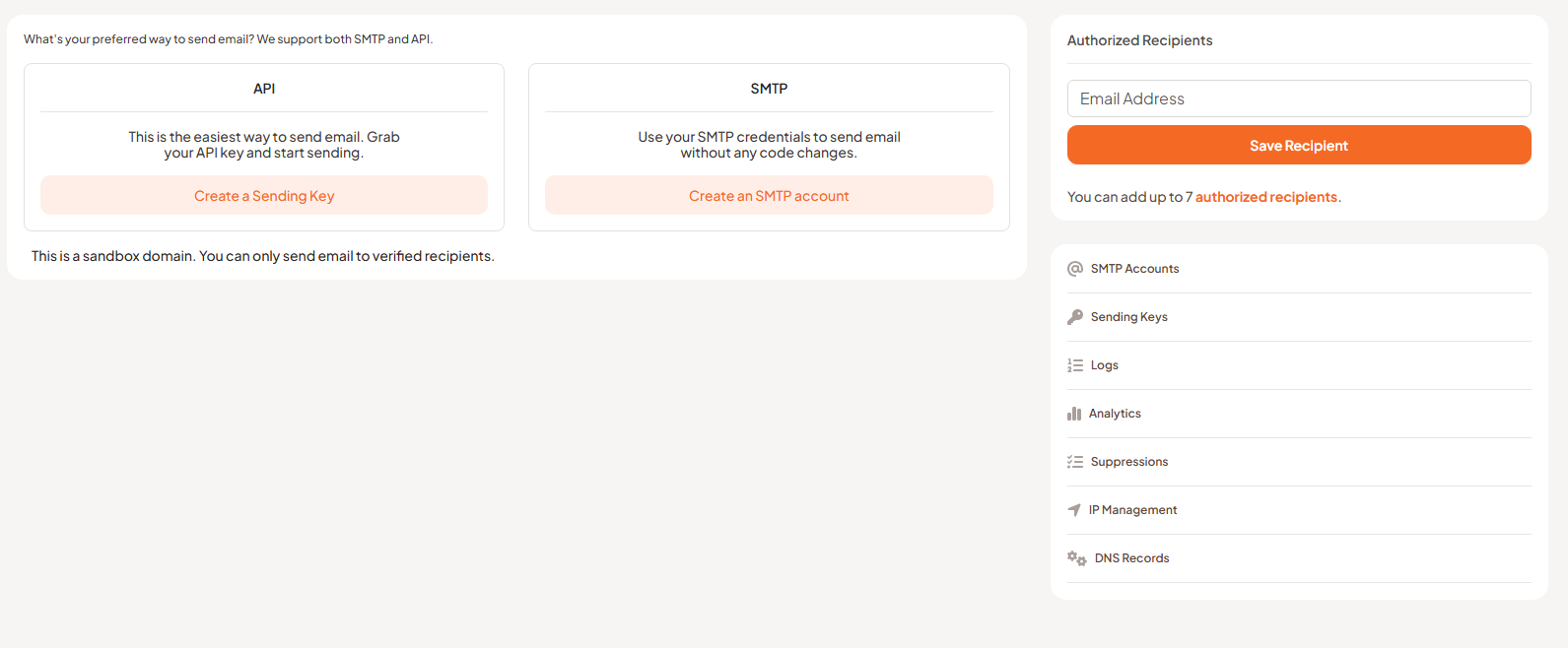
With your environment set up ready and Maileroo account, let's start working on the email automation project!
1. Sending Emails Using SMTP
Before we send the email via SMTP, we will need to fill in our SMTP credentials.
- SMTP Username: Your Maileroo email address
- SMTP Password: Your Maileroo password
Based on these, here's a simple script to send email (e.g. send_smtp_email.py)
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
# SMTP Configuration
SMTP_HOST = 'smtp.maileroo.com'
SMTP_PORT = 587
SMTP_USERNAME = '[email protected]' # Replace with your email address
SMTP_PASSWORD = 'your_password' # Replace with your email password
SENDER_NAME = 'Your Name' # Replace with your sender name
# Email Details
RECIPIENTS = ['[email protected]', '[email protected]'] # Replace with recipient email addresses
SUBJECT = 'Sending Email Using SMTP'
MESSAGE_BODY = 'This is a test email sent using the SMTP server.'
def send_email():
# Create Email Message
message = MIMEMultipart()
message['From'] = f'{SENDER_NAME} <{SMTP_USERNAME}>'
message['To'] = ', '.join(RECIPIENTS)
message['Subject'] = SUBJECT
message.attach(MIMEText(MESSAGE_BODY, 'plain'))
# Send Email
try:
with smtplib.SMTP(SMTP_HOST, SMTP_PORT) as server:
server.starttls() # Secure the connection
server.login(SMTP_USERNAME, SMTP_PASSWORD)
server.sendmail(SMTP_USERNAME, RECIPIENTS, message.as_string())
print("Email sent successfully!")
except Exception as e:
print(f"Failed to send email: {e}")
if __name__ == "__main__":
send_email()
And done, now let's save the code and execute the script:
python send_smtp_email.py
Send HTML email
To send HTML emails, we just change the body to html
:
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
# SMTP Configuration
SMTP_HOST = 'smtp.maileroo.com'
SMTP_PORT = 587
SMTP_USERNAME = '[email protected]' # Replace with your email address
SMTP_PASSWORD = 'your_password' # Replace with your email password
SENDER_NAME = 'Your Name' # Replace with your sender name
# Email Details
RECIPIENTS = ['[email protected]', '[email protected]'] # Replace with recipient email addresses
SUBJECT = 'Sending Email Using SMTP'
MESSAGE_BODY = '''
<h1>Good Morning!</h1>
<p>This is a test email sent via <strong>SMTP</strong>.</p>
'''
def send_email():
# Create Email Message
message = MIMEMultipart()
message['From'] = f'{SENDER_NAME} <{SMTP_USERNAME}>'
message['To'] = ', '.join(RECIPIENTS)
message['Subject'] = SUBJECT
message.attach(MIMEText(MESSAGE_BODY, 'html'))
# Send Email
try:
with smtplib.SMTP(SMTP_HOST, SMTP_PORT) as server:
server.starttls() # Secure the connection
server.login(SMTP_USERNAME, SMTP_PASSWORD)
server.sendmail(SMTP_USERNAME, RECIPIENTS, message.as_string())
print("Email sent successfully!")
except Exception as e:
print(f"Failed to send email: {e}")
if __name__ == "__main__":
send_email()
2. Sending Emails Using Maileroo API
The Maileroo API is a powerful RESTful tool that simplifies email delivery, offering advanced features like tracking, template management, and high deliverability. It’s an excellent choice for developers looking to build reliable and scalable email workflows efficiently.
Make sure to create a Maileroo account, as you’ll need an API key to get started!
Sending Plain Text Emails
Here's a script to send plain text emails using the Maileroo API (eg: send_email_api.py
):
import requests
# API Configuration
API_KEY = 'your-api-key-here' # Replace with your Maileroo API Key
API_URL = 'https://smtp.example.com/send'
# Email Details
SENDER_EMAIL = '[email protected]' # Replace with your email address
SENDER_NAME = 'Your Name' # Replace with your name
RECIPIENT_EMAIL = '[email protected]' # Replace with recipient's email
SUBJECT = 'Hello from the Email API!'
MESSAGE_BODY = 'This is a test email sent using the Maileroo Email API.'
def send_email():
"""Send an email using a third-party API."""
# Email Payload
payload = {
'from': f'{SENDER_NAME} <{SENDER_EMAIL}>',
'to': RECIPIENT_EMAIL,
'subject': SUBJECT,
'plain': MESSAGE_BODY,
}
try:
# API Request
response = requests.post(API_URL, headers={'X-API-Key': API_KEY}, data=payload)
if response.status_code == 200 and response.json().get('success'):
print("Email sent successfully!")
else:
error_message = response.json().get('message', 'Unknown error')
print(f"Failed to send email: {error_message}")
except Exception as e:
print(f"Error: {e}")
if __name__ == "__main__":
send_email()
Save and run:
python send_email_api.py
Customizing the Email Name
You can also customize the sender’s name and format it in different ways:
[email protected]
"Name" <[email protected]>
<[email protected]>
Update the from
field in the payload with the new format.
Sending HTML Emails
To send HTML emails, just update the payload to include an html
field:
payload = {
'from': SENDER_EMAIL,
'to': RECIPIENT_EMAIL,
'subject': SUBJECT,
'html': '''
<h1>Welcome!</h1>
<p>This is a test email sent using <strong>Maileroo API</strong>.</p>
''',
}
Using Templates
Maileroo also allows you to create templates with dynamic placeholders for sending personalized emails.
Here’s the code to send an email using a template:
payload = {
'from': sender,
'to': recipient,
'subject': subject,
'template_id': 'your-template-id', # Replace with your Template ID
'template_data': '{"name": "John Doe"}', # Replace with your dynamic data
}
Conclusion
In this article, we've explored how Python can be used to send emails in different ways. We hope this helps you in developing email sending functionality in your app!
While SMTP offers simplicity for basic email delivery, the Maileroo API stands out with its flexibility, scalability, and enhanced features like tracking and template management, now take the time to choose the method that best suits your project’s needs.
You can also visit our official Maileroo Documentation for more in-depth details.
FAQs
1. Can I send attachments using Maileroo?
Absolutely! Maileroo supports sending attachments. Simply include the attachments
field in your API payload with the file details, such as name, type, and content. You can also check our Email Sending API Documentation for detailed instructions.
2. How can I troubleshoot email delivery issues?
Maileroo provides a comprehensive dashboard with detailed logs and analytics for every email sent. You can easily identify issues by reviewing delivery statuses, error codes, and engagement metrics in the Maileroo | Statistics.
3. Is there a limit to the number of emails I can send?
Yes, Maileroo offers a monthly limit of 5,000 emails under its free plan. For higher limits or business requirements, you can explore our pricing plans.
4. What authentication methods does Maileroo support?
Maileroo uses API keys for secure authentication. You can generate and manage your keys directly from the dashboard.
6. Are there any Python libraries to simplify integration?
Maileroo's REST API works seamlessly with Python's requests
library, but other options like yagmail
or flask-mail
can be used depending on your project requirements.
7. Can I send bulk emails using the API?
Yes, Maileroo supports bulk email sending. You can include multiple recipients in the to
field for large-scale campaigns. Don't forget to add the recipients to your list of Authorized Recipients.