How to Send Emails in Node.js: Nodemailer and HTTP API
Learn to send emails in Node.js with Maileroo's SMTP and API. This guide covers setup, using Nodemailer, sending plain text, HTML, and template emails, and securing API keys. Streamline email delivery with these easy steps.
Emails are at the heart of modern applications—whether you're sending notifications, handling password resets, or running promotional campaigns. In this guide, we’ll take a hands-on, step-by-step approach to explore how to send emails using Node.js. Specifically, we’ll focus on exploring these two methods, sending emails using SMTP and using Maileroo Email API.
For both approaches, we’ll be using Maileroo, a user-friendly, affordable, and easy-to-use platform. Along the way, we’ll not only cover the code but also the setup process, including how to create a Maileroo account and configure everything you need to get started.
What is Maileroo?
Maileroo is an email delivery platform specializing in streamlining and automating email processes for individuals, small businesses, and growing companies. It's a full-service email marketing company focusing on transactional email delivery.
Maileroo’s email delivery API offers stress-free and reliable transactional email sending. The API easily integrates into any application or website and supports SMTP, offering a quick way to start sending transaction emails from a web application. Communications are encrypted in transit, and the platform relies on 2FA for an additional layer of security.
Getting Started
First, let's register a free Maileroo account and verifying your domain. This process only takes a couple of minutes and is very simple.
- After signing up, you’ll receive a verification email. Click the link in your email to verify your account.
- Once verified, log in to your account.
- From the dashboard, choose SMTP Relay to begin.
Creating an SMTP Account
To send emails using SMTP, we first need to set up the necessary credentials.
1. Go to Domains and click on Overview.
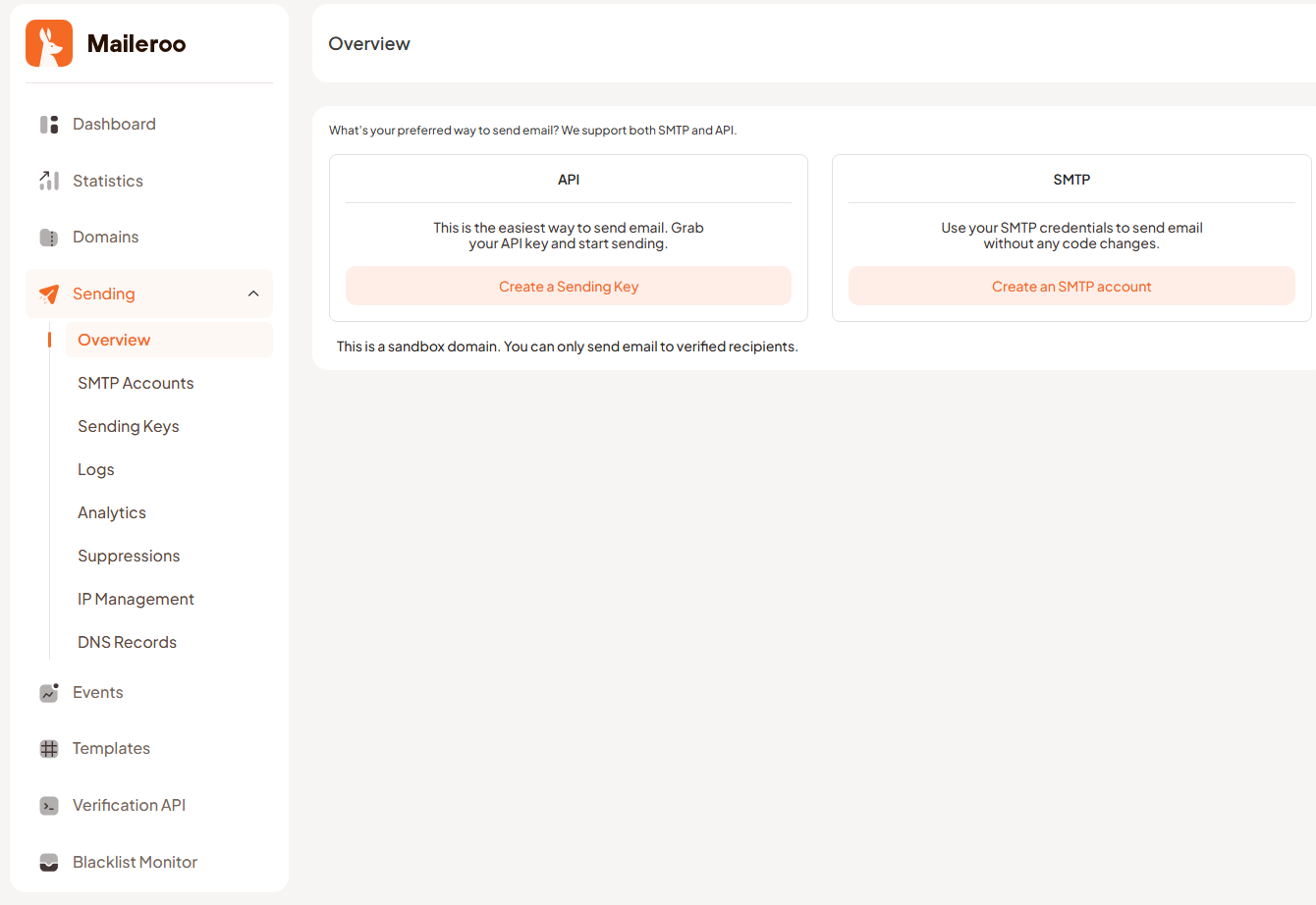
2. Click on Create an SMTP Account and then New Account
3. Add an alias for the email address you’ll use to send emails.
That's it, your SMTP credentials are now ready to use.
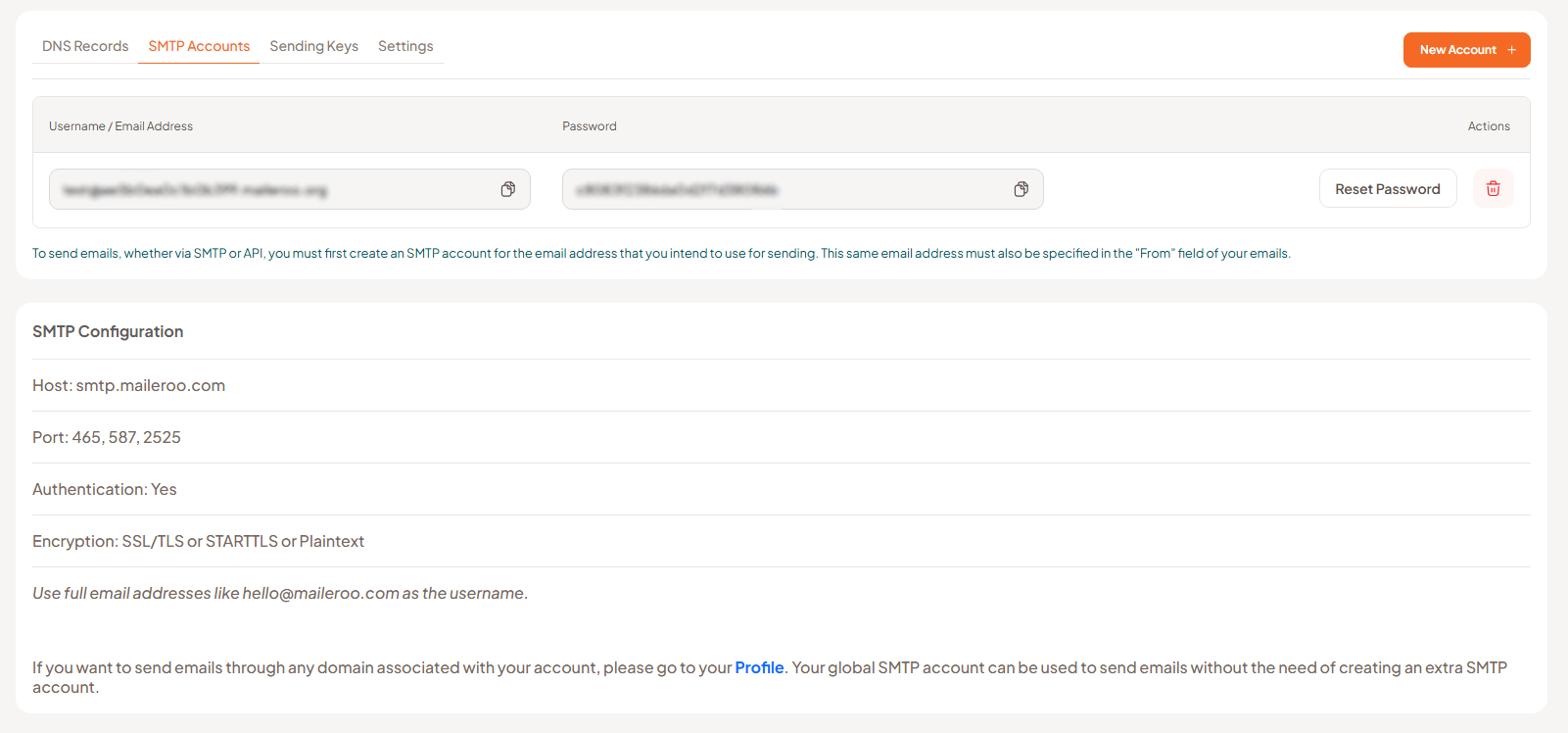
Creating a Sending Key for API Use
If you prefer to send emails using the Maileroo Email API, you’ll need an API key for authentication. Here’s how to create it:
- Navigate to Overview in your domain settings and click on Create a Sending Key, then New Sending Key.
- Provide an identifier (you can use any name you like).

We can now use this API key to send emails.
Sending Email Using SMTP
SMTP (Simple Mail Transfer Protocol) is the standard protocol used for sending emails between various servers. It’s the backbone of email communication, allowing services like Gmail, Outlook, and Yahoo to send messages efficiently.
In this guide, we’ll use Nodemailer, a popular Node.js library that simplifies working with SMTP. It simplifies the process of integrating SMTP with your app and it's very simple to use.
Let's start by creating a Node.js application
mkdir smtp-mailapp && cd smtp-mailapp
npm init -y
Now we've created our folder and initilized the package.json
file with the npm init
. The -y
flag is used to populate all the options automatically with the default npm init values.
Next, let's install the nodemailer package:
npm install nodemailer
To send emails via SMTP, we need to configure a transporter in Nodemailer using the createTransport
method. This transporter will include the connection details for the Maileroo SMTP server.
Here's a sample code which you can directly paste in your file (e.g. index.js):
const nodemailer = require('nodemailer');
// SMTP configuration
const SMTP_HOST = 'smtp.maileroo.com';
const SMTP_PORT = 587;
const SMTP_USERNAME = 'your-email@maileroo.org'; // Replace with your SMTP username
const SMTP_PASSWORD = 'your-smtp-password'; // Replace with your SMTP password
const SMTP_SENDER_NAME = 'Your Name'; // Replace with your preferred sender name
// Recipient email
const receiverEmail = 'recipient@example.com'; // Replace with the recipient's email
const transporter = nodemailer.createTransport({
host: SMTP_HOST,
port: SMTP_PORT,
auth: {
user: SMTP_USERNAME,
pass: SMTP_PASSWORD,
},
secure: false,
requireTLS: true,
});
const mailOptions = {
from: `${SMTP_SENDER_NAME} <${SMTP_USERNAME}>`,
to: receiverEmail,
subject: 'Sending Email using SMTP',
text: 'Hey! This is a sample email sent using the Maileroo SMTP server.',
};
transporter.sendMail(mailOptions, (error, info) => {
if (error) {
console.error(`Failed to send email. Error: ${error.message}`);
} else {
console.log('Email sent successfully:', info);
}
});
Now before running the code, let's replace the configuration variables with actual values,
- SMTP_HOST: The Maileroo SMTP server address (default:
smtp.maileroo.com
). - SMTP_PORT: This is the port for connecting to the SMTP server. We can use any of the following,
465, 587, 2525
. - SMTP_USERNAME: Your Maileroo SMTP username (found in your Maileroo account under SMTP Accounts).
- SMTP_PASSWORD: Your Maileroo SMTP password (also available in the SMTP Accounts section).
- SMTP_SENDER_NAME: The sender name that will appear in the email
The username and password can be found on your Maileroo's SMTP Accounts.
You can also refer to the official Maileroo documentation for detailed SMTP setup: SMTP Details | Maileroo.
And, that's it! Let's save the file and run the script, with the following command:
node index.js
Tip: If you're using our sandbox domain, don't forget to add the recipient email in Authorized Recipient List.
For advanced mail configuration options, check out the Nodemailer Message Configuration Guide.
Sending Email Using Maileroo API
In addition to SMTP, Maileroo also provides an Email API for sending emails directly. This approach offers flexibility and is especially useful for developers who prefer API-based integration over SMTP.
Before we begin, ensure you have already created an SMTP account in your Maileroo dashboard. The email address used for the API will be the same as the one linked to your SMTP account. Refer to the Creating SMTP Account section for more details.
We will also need Maileroo Sending Key for authentication.
Let's start by setting up our project and installing packages:
mkdir maileroo-mailapp && cd maileroo-mailapp
npm init -y
Send a plain text email
Let's use the following code to start sending emails, (e.g. index.js)
// Your Maileroo API key
const api_key = 'your-maileroo-api-key'; // Replace with your actual API key
async function sendEmail() {
const formData = new FormData();
// Add email details
formData.append('from', 'Your Name <your-email@maileroo.com>'); // Replace with sender email
formData.append('to', 'recipient@example.com'); // Replace with recipient email
formData.append('subject', 'Hello from Maileroo API!');
formData.append('plain', 'This is a plain text email via the Maileroo API.');
try {
const response = await fetch('https://smtp.maileroo.com/send', {
method: 'POST',
headers: {
'X-API-Key': api_key,
},
body: formData,
});
const result = await response.json();
if (response.ok && result.success) {
console.log('Email sent successfully:', result.message);
} else {
console.error('Error sending email:', result.message);
}
} catch (error) {
console.error('Error sending email:', error.response?.data || error.message);
}
}
sendEmail();
Now just save and run it
node index.js
That's it, we successfully sent our first email using the API.
You’ll find more examples further below, but for more in-depth details and advanced use cases, refer to the Email Sending API Documentation.
Note: You can send email using either plain or html but at least one of them is required.
Send an HTML email
To send an email with HTML content, we just have to change the plain text into html text, like this:
formData.append('html', `
<h2>HTML Email via Maileroo API</h2>
<p>This is a sample email sent using the Maileroo API.</p>
<p>Enjoy sending emails effortlessly!</p>
`);
Customizing the Email Name
You can also customize the sender’s name and format it in different ways:
example@maileroo.com
"Name" <example@maileroo.com>
<example@maileroo.com>
Update the from
field in your email data with the new format.
Send email to multiple recipients
The Maileroo API supports sending emails to multiple recipients in a single request. To include multiple recipients, use the cc
field as follows:
formData.append('cc', 'firstEmail@gmail.com, secondEmail@gmail.com');
This will send a copy of the email to the additional recipients specified. Don't forget to add them to your authorized recipients list.
Send an Email Using Templates
Maileroo allows you to send customized, personalized emails to multiple recipients at once using templates. This feature is especially useful for mass emailing while maintaining a personal touch for each recipient.
Let's start by creating a template in the Maileroo Templates section. Click on Create Template, and you’ll be presented with an intuitive editor that lets you design your email and preview it simultaneously.
To personalize the email, you can include tags in your template, like {{name}}
. These tags act as placeholders that are automatically replaced with the data you provide in your API request.
Tip: Ensure there are no spaces within the curly braces (e.g., {{name}}
). Tags with spaces won’t work.
Once your template is complete, save it, and copy the Template ID displayed below your template for use in the API request.
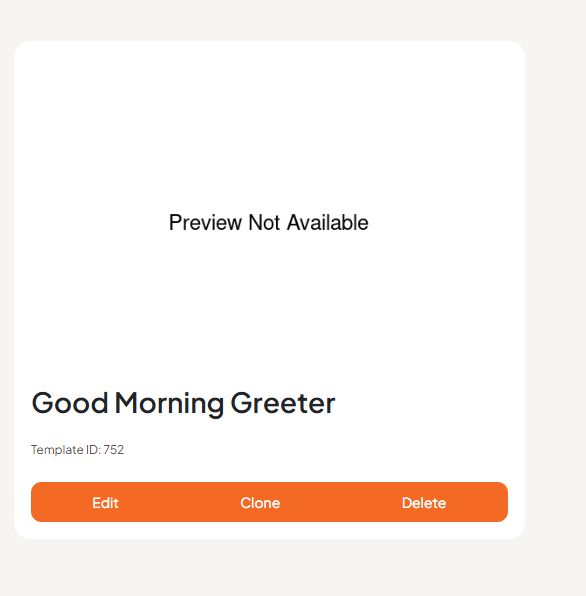
Now let's use the following code to send the email (save it as template.js):
// Your Maileroo API key
const api_key = 'your-maileroo-api-key'; // Replace with your actual API key
async function sendTemplateEmail() {
const formData = new FormData();
// Add template email details
formData.append('from', 'Your Name <your-email@maileroo.com>'); // Replace with sender email
formData.append('to', 'recipient@example.com'); // Replace with recipient email
formData.append('subject', 'Welcome to Maileroo!');
formData.append('template_id', '752'); // Replace with your template ID
formData.append(
'template_data',
JSON.stringify({
name: 'Areeb!', // Replace with dynamic data
})
);
try {
const response = await fetch('https://smtp.maileroo.com/send-template', {
method: 'POST',
headers: {
'X-API-Key': api_key,
},
body: formData,
});
const result = await response.json();
if (response.ok && result.success) {
console.log('Email sent successfully:', result.message);
} else {
console.error('Error sending email:', result.message);
}
} catch (error) {
console.error('Error sending template email:', error.response?.data || error.message);
}
}
sendTemplateEmail();
Now let's just save it and run
node template.js
We can now send personalized email using Maileroo!
Secure API Key Storage
Hardcoding your API key directly into the script is not secure. Here’s another method to securely store and access your API key using environment variables:
Firstly let's create a .env
file in our project directory and add the API key there:
MAILEROO_API_KEY=your-maileroo-api-key
Install the dotenv
package:
npm install dotenv
In the script add the following code to load the API key from the environment file:
import dotenv from 'dotenv';
dotenv.config();
const api_key = process.env.MAILEROO_API_KEY;
That's it, now we can use the updated api_key
variable in the script instead of hardcoding it.
Tip: If you plan to share your code publicly, ensure you add the.env
file to your.gitignore
to keep your sensitive credentials secure.
Wrap Up
So this was all about how to send emails using NodeJS.
You’ve learned how to send emails using both SMTP and Maileroo Email API. We hope this guide made it easy to understand and helped you get started with email sending.